Hooking stride
The minimum byte-distance between two member layouts is called stride. The stride can be greater than or equal to the size. When we don’t face any alignment issues the stride is equal to the size. Otherwise, the stride is computed by rounding up the size to the next multiple of the alignment. When the stride is greater than the size it means that we also have some padding. If we have a C-like struct/union named foo then stride is the minimum byte-distance between two foo objects.
Hooking padding
So, padding is the amount of extra space that we need to add in order to preserve a valid alignment of the member layouts.Don’t worry if you are a little bit confused about all these statements. We will clarify everything via a bunch of examples.
Adding implicit extra space (implicit padding) to validate alignment
Let’s consider the following simple example:
MemorySegment segment = MemorySegment
.allocateNative(12, SegmentScope.auto());
segment.set(ValueLayout.JAVA_INT, 0, 1000);
segment.set(ValueLayout.JAVA_CHAR, 4, ‘a’);
We have a memory segment of 12 bytes and we have set an int of 4 bytes at offset 0 and a char of 2 bytes at offset 4 (immediately after the int). So, we still have 6 free bytes. Let’s assume that we want to set one more int of 4 bytes after the char, a. What should be the offset? Based on the first thought, we may think that the proper offset is 6 since the char consumed 2 bytes:
segment.set(ValueLayout.JAVA_INT, 6, 2000);
But, if we do this then the result is java.lang.IllegalArgumentException: Misaligned access at the address: …. We have a misaligned member layout (the 2000 int value) because 6 is not evenly divisible by 4 which is the byte alignment of int. Check out the following figure:
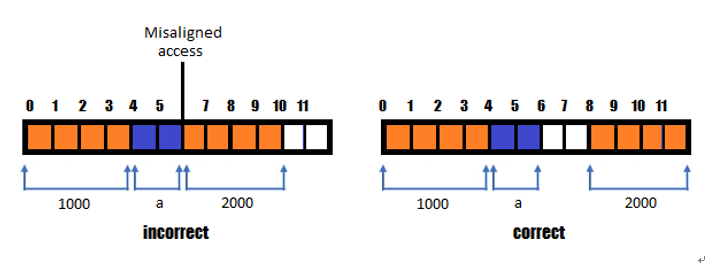
Figure 7.16 – Fixing the misaligned issue
But, how we should think? We know that the current offset is 6 and 6 is not evenly divisible by 4 (the int alignment). So, we are looking for the next offset that is evenly divisible by 4 and is the closest and greater than 6. Obviously, this is 8. So, before we set the 2000 int value, we need a padding of 2 bytes (16 bits). This padding will be automatically added if we simply specify the offset 8 instead of 6:
segment.set(ValueLayout.JAVA_INT, 8, 2000);
Since our memory segment has a size of 12 bytes, we fit this int exactly on bytes 8, 9, 10, and 11. A smaller size of the segment leads to an IndexOutOfBoundsException: Out of bound access on segment MemorySegment.